8.7 CÁC TRẠNG THÁI ĐỊNH DẠNG D̉NG
Các cờ định dạng khác nhau mô tả các loại định dạng được thực thi trong suốt quá trình thao tác nhâp/xuất dòng. Các hàm ios::setf(), ios::unsetf(), và ios::flags() điều khiển các thiết lập cờ.
Hàm ios::setf():
(1) long setf(long lFlags);
(2) long setf(long lFlags, long lMask);
Trong đó:
lFlags: Định dạng các giá trị bit cờ. Có thể kết hợp các bit cờ bằng cách sử dụng toán tử |. Các bit cờ:
Bit cờ |
Ý nghĩa |
ios::skipws |
Bỏ qua khoảng trắng trên nhập. |
ios::left |
Giá trị canh lề trái; Độn thêm bên phải với ký tự lấp đầy. |
ios::right |
Giá trị canh lề phải; Độn thêm bên trái với ký tự lấp đầy (mặc định). |
ios::internal |
Thêm các ký tự lấp đầy sau bất kỳ dấu chỉ dẫn hoặc dấu hiệu cơ số nào, nhưng trước giá trị. |
ios::dec |
Giá trị số định dạng theo cơ số 10 (mặc định). |
ios::oct |
Giá trị số định dạng theo cơ số 8. |
ios::hex |
Giá trị số định dạng theo cơ số 16. |
ios::showbase |
Hiển thị các hằng số theo dạng mà có thể đọc bởi trình biên dịch C++. |
ios::showpoint |
Xuất hiện dấu thập phân và dạng thức zero theo đuôi cho các giá trị chấm động. |
ios::uppercase |
Hiển thị chữ hoa từ A đến F đối với giá trị hệ 16 và E đối với các giá trị dạng khoa học. |
ios::showpos |
Hiển thị dấu + đối với các số dương. |
ios::scientific |
Hiển thị số chấm động theo dạng khoa học. |
ios::fixed |
Hiển thị số chấm động theo dạng cố định |
lMask: Định dạng mặt nạ bit cờ.
Hàm trả về giá trị các bit cờ trước đó.
Hàm ios::flags():
(1) long flags(long lFlags);
(2) long flags();
Dạng (2) trả về các bit cờ hiện tại của dòng. Dạng (1) giống như dạng (1) của hàm ios::setf().
Hàm ios::unsetf():
long unsetf(long lFlags);
Hàm xóa các bit cờ chỉ định bởi lFlags.
Ngoài ra chúng ta còn có hàm setiosflags() giống như dạng (1) của ios::setf() và hàm resetiosflags() giống như hàm unsetf().
Các cờ bit ios::left, ios::right,và ios::internal được chứa trong biến thành viên ios::adjustfield. Chẳng hạn:
ostream os;
if( ( os.flags() & ios::adjustfield ) == ios::left )
.....
Tham số ios::adjustfield phải được cung cấp như tham số thứ 2 ở dạng (2) của hàm ios::setf() khi ấn định các cờ bit căn lề (ios::left, ios::right,và ios::internal). Điều này cho phép ios::setf() bảo đảm chỉ có một trong ba cờ bit căn lề được ấn định. Chẳng hạn:
cout.setf(ios::left,ios::adjustfield);
Ví dụ 8.18: Cờ bit ios::showpoint.
 |
 |
CT8_18.CPP
|
 |
|
1: //Chương trình 8.18
2: #include <iostream.h>
3: #include <iomanip.h>
4: #include <math.h>
5:
6: int main()
7: {
8: cout << "cout prints 9.9900 as: " << 9.9900 << endl
9: << "cout prints 9.9000 as: " << 9.9000 << endl
10: << "cout prints 9.0000 as: " << 9.0000 << endl << endl
11: << "After setting the ios::showpoint flag" << endl;
12: cout.setf(ios::showpoint);
13: cout << "cout prints 9.9900 as: " << 9.9900 << endl
14: << "cout prints 9.9000 as: " << 9.9000 << endl
15: << "cout prints 9.0000 as: " << 9.0000 << endl;
16: return 0;
17: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.18, kết quả ở hình 8.20

Hình 8.20: Kết quả của ví dụ 8.18
Ví dụ 8.19: Cờ bit ios::left, ios::right, ios::internal.
 |
 |
CT8_19.CPP
|
 |
|
1: //Chương trình 8.19
2: #include <iostream.h>
3: #include <iomanip.h>
4:
5: int main()
6: {
7: int X = 12345;
8: cout << "Default is right justified:" << endl
9: << setw(10) << X << endl << endl
10: << "USING MEMBER FUNCTIONS" << endl
11: << "Use setf to set ios::left:" << endl << setw(10);
12: cout.setf(ios::left, ios::adjustfield);
13: cout << X << endl << "Use unsetf to restore default:" << endl;
14: cout.unsetf(ios::left);
15: cout << setw(10) << X << endl << endl
16: << "USING PARAMETERIZED STREAM MANIPULATORS" << endl
17: << "Use setiosflags to set ios::left:" << endl
18: << setw(10) << setiosflags(ios::left) << X << endl
19: << "Use resetiosflags to restore default:" << endl
20: << setw(10) << resetiosflags(ios::left)
21: << X << endl;
22: return 0;
23: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.19, kết quả ở hình 8.21
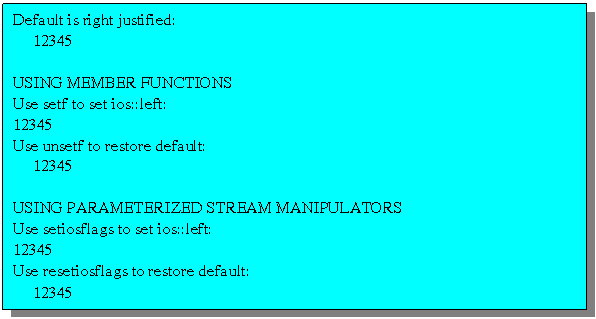
Hình 8.21: Kết quả của ví dụ 8.19
Ví dụ 8.20: Cờ bit ios::showpos và ios::internal.
 |
 |
CT8_20.CPP
|
 |
|
1: //Chương trình 8.20
2: #include <iostream.h>
3: #include <iomanip.h>
4:
5: int main()
6: {
7: cout << setiosflags(ios::internal | ios::showpos)
8: << setw(10) << 123 << endl;
9: return 0;
10: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.20, kết quả ở hình 8.22

Hình 8.22: Kết quả của ví dụ 8.20
Đ
ể ký tự lấp đầy chúng ta dùng hàm ios::fill() và setfill():
Hàm ios::fill():
(1) char fill(char cFill);
(2) char fill();
Dạng (1) ấn định ký tự lấp đầy nội tại và trả về ký tự lấp đầy trước đó. Dạng (2) trả về ký tự lấp đầy của dòng.
Hàm setfill():
SMANIP(int) setfill(int nFill);
Ví dụ 8.21: Hàm ios::fill() và setfill().
 |
 |
CT8_21.CPP
|
 |
|
1: //Chương trình 8.21
2: #include <iostream.h>
3: #include <iomanip.h>
4:
5: int main()
6: {
7: int X = 10000;
8: cout << X
9: << " printed as int right and left justified" << endl
10: << "and as hex with internal justification." << endl
11: << "Using the default pad character (space):" << endl;
12: cout.setf(ios::showbase);
13: cout << setw(10) << X << endl;
14: cout.setf(ios::left, ios::adjustfield);
15: cout << setw(10) << X << endl;
16: cout.setf(ios::internal, ios::adjustfield);
17: cout << setw(10) << hex << X << endl << endl;
18: cout << "Using various padding characters:" << endl;
19: cout.setf(ios::right, ios::adjustfield);
20: cout.fill('*');
21: cout << setw(10) << dec << X << endl;
22: cout.setf(ios::left, ios::adjustfield);
23: cout << setw(10) << setfill('%') << X << endl;
24: cout.setf(ios::internal, ios::adjustfield);
25: cout << setw(10) << setfill('^') << hex
26: << X << endl;
27: return 0;
28: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.21, kết quả ở hình 8.23
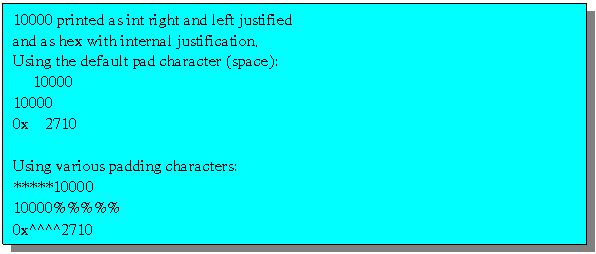
Hình 8.23: Kết quả của ví dụ 8.21
Ví dụ 8.22: Cờ bit ios::showbase.
 |
 |
CT8_22.CPP
|
 |
|
1: //Chương trình 8.22
2: #include <iostream.h>
3: #include <iomanip.h>
4:
5: int main()
6: {
7: int X = 100;
8: cout << setiosflags(ios::showbase)
9: << "Printing integers preceded by their base:" << endl
10: << X << endl
11: << oct << X << endl
12: << hex << X << endl;
13: return 0;
14: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.22, kết quả ở hình 8.24

Hình 8.24: Kết quả của ví dụ 8.22
Ví dụ 8.23: Hiển thị các giá trị chấm động theo dạng mặc định hệ thống, dạng khoa học và dạng cố định.
 |
 |
CT8_23.CPP
|
 |
|
1: //Chương trình 8.23
2: #include <iostream.h>
3:
4: int main()
5: {
6: double X = .001234567, Y = 1.946e
7: cout << "Displayed in default format:" << endl
8: << X << '\t' << Y << endl;
9: cout.setf(ios::scientific, ios::floatfield);
10: cout << "Displayed in scientific format:" << endl
11: << X << '\t' << Y << endl;
12: cout.unsetf(ios::scientific);
13: cout << "Displayed in default format after unsetf:" << endl
14: << X << '\t' << Y << endl;
15: cout.setf(ios::fixed, ios::floatfield);
16: cout << "Displayed in fixed format:" << endl
17: << X << '\t' << Y << endl;
18: return 0;
19: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ
8.23, kết quả ở hình 8.25
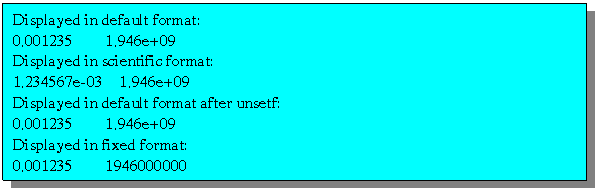
Hình 8.25: Kết quả của ví dụ 8.23
Ví dụ 8.24: Cờ bit ios::uppercase.
 |
 |
CT8_24.CPP
|
 |
|
1: //Chương trình 8.24
2: #include <iostream.h>
3: #include <iomanip.h>
4:
5: int main()
6: {
7: cout << setiosflags(ios::uppercase)
8: << "Printing uppercase letters in scientific" << endl
9: << "notation exponents and hexadecimal values:" << endl
10: << 4.345e10 << endl
11: << hex << 123456789 << endl;
12: return 0;
13: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.24, kết quả ở hình 8.26

Hình 8.26: Kết quả của ví dụ 8.24
Ví dụ 8.25: Ấn định và xác lập lại các cờ định dạng (ios::flags, setiosflags(), resetiosflags()).
 |
 |
CT8_25.CPP
|
 |
|
1: //Chương trình 8.25
2: #include <iostream.h>
3: int main()
4: {
5: int I = 1000;
6: double D = 0.0947628;
7: cout << "The value of the flags variable is: "
8: << cout.flags() << endl
9: << "Print int and double in original format:" << endl
10: << I << '\t' << D << endl << endl;
11: long originalFormat = cout.flags(ios::oct | ios::scientific);
12: cout << "The value of the flags variable is: "
13: << cout.flags() << endl
14: << "Print int and double in a new format" << endl
15: << "specified using the flags member function:" << endl
16: << I << '\t' << D << endl << endl;
17: cout.flags(originalFormat);
18: cout << "The value of the flags variable is: "
19: << cout.flags() << endl
20: << "Print values in original format again:" << endl
21: << I << '\t' << D << endl;
22: return 0;
23: }
|
 |
|
|
 |
|
|
 |
Chúng ta chạy ví dụ 8.25, kết quả ở hình 8.27
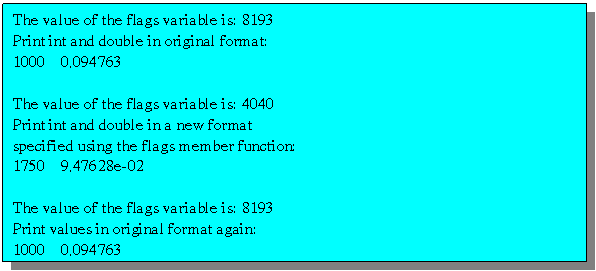
Hình 8.27: Kết quả của ví dụ 8.25